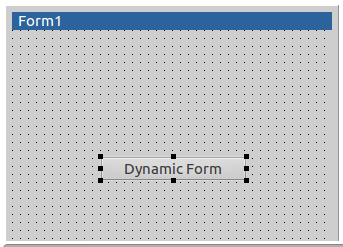
Just click on File | New … and a Form opens up. Select Project | Application and Unit1 – Form1 shows up in the editor. Add a TButton from the Standard Tab to the current Form1.
Edit Caption attribute from the just created Button1 element and change it to “Dynamic Form” (or what ever you want to name it).
Double click on the Button1 and the event editor will show up with the current code:
procedure TForm1.Button1Click(Sender: TObject);
beginend;
Add the following code to this event handler:
procedure TForm1.Button1Click(Sender: TObject);
var
MyForm: TForm;
MyButton: TButton;
begin
//Create MyForm
MyForm:=TForm.Create(nil);
//Set position and attributes
MyForm.SetBounds(100, 100, 220, 150);
MyForm.Caption:=’My dynamic created form’;//Create Button
MyButton:=TButton.create(MyForm);
//Set size and attributes
MyButton.Caption:=’Close my form’;
MyButton.SetBounds(10, 10, 200, 30);
//Assign to parent form
MyButton.Parent:=MyForm;
//Event handler pointer to our procedure above…
MyButton.OnClick:=@MyButtonClick;
//Show My Wonderful Dynamic Form
MyForm.ShowModal;//Destroy & Free all memory from it
FreeAndNil(MyForm);
end;
Also add just before this event handler, and after {$R *.frm} { TForm1 } the following function:
//Method/Event to close the dynamic form
procedure TForm1.MyButtonClick(Sender: TObject);
begin
//Just make sure who called us
if Sender is TButton then
begin
//warning you are about to close your wonderful form!!
Showmessage(‘Attention, my dynamically created form is closed!’);
TForm(TButton(Sender).Parent).Close;
end;
end;
Add the following in the TForm1 declaration after procedure Button1Click(Sender: TObject);
procedure MyButtonClick(Sender: TObject);
Compile and run.